Sorting Technology
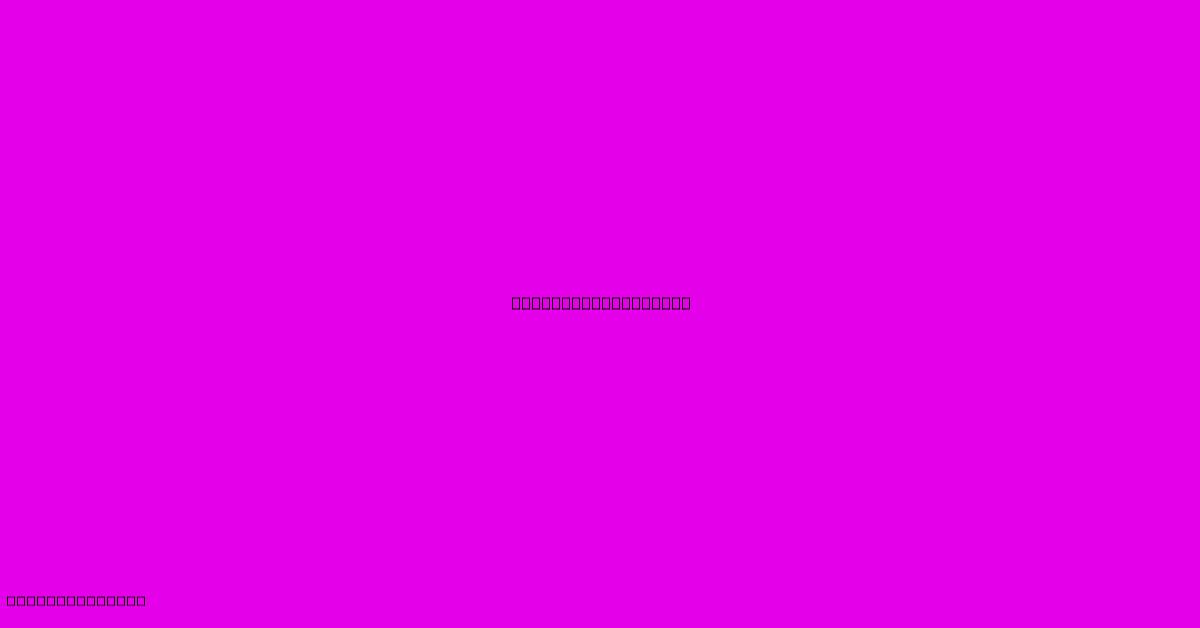
Discover more detailed and exciting information on our website. Click the link below to start your adventure: Visit Best Website mr.cleine.com. Don't miss out!
Table of Contents
Sorting Technology: A Deep Dive into Algorithms and Applications
Sorting, the process of arranging items in a specific order (e.g., numerical, alphabetical), is a fundamental operation in computer science. Efficient sorting is crucial for numerous applications, impacting everything from database searches to artificial intelligence algorithms. This article explores various sorting technologies, their complexities, and real-world implications.
Understanding Sorting Algorithms:
The choice of sorting algorithm depends heavily on factors like the size of the data set, the nature of the data (e.g., nearly sorted, random), and the available memory. Here are some prominent algorithms:
1. Bubble Sort:
- Mechanism: Repeatedly steps through the list, compares adjacent elements, and swaps them if they are in the wrong order. The pass through the list is repeated until no swaps are needed, indicating that the list is sorted.
- Complexity: O(n²) – inefficient for large datasets. Simple to understand and implement.
- Use Cases: Suitable for small datasets or educational purposes due to its simplicity.
2. Insertion Sort:
- Mechanism: Builds the final sorted array one item at a time. It iterates through the input, picking one element and inserting it into its correct position within the already sorted part of the array.
- Complexity: O(n²) in the worst and average cases, O(n) in the best case (already sorted). Efficient for small datasets or nearly sorted data.
- Use Cases: Good for small datasets or when the data is nearly sorted. Often used as a subroutine in other algorithms like quicksort (hybrid approaches).
3. Selection Sort:
- Mechanism: Repeatedly finds the minimum element from the unsorted part and puts it at the beginning. The algorithm maintains two subarrays in a given array.
- Complexity: O(n²) – inefficient for large datasets.
- Use Cases: Simple to implement, but not efficient for large datasets.
4. Merge Sort:
- Mechanism: A divide-and-conquer algorithm that recursively divides the unsorted list into smaller sublists until each sublist contains only one element (a list of one element is considered sorted). Then it repeatedly merges the sublists to produce new sorted sublists until there is only one sorted list remaining.
- Complexity: O(n log n) – efficient for large datasets. Stable sort (preserves the relative order of equal elements).
- Use Cases: Excellent for large datasets where efficiency is paramount. Used in external sorting (data too large to fit in memory).
5. Quicksort:
- Mechanism: Another divide-and-conquer algorithm. Selects a 'pivot' element and partitions the other elements into two sub-arrays, according to whether they are less than or greater than the pivot. The sub-arrays are then recursively sorted.
- Complexity: Average case O(n log n), worst case O(n²) (e.g., already sorted data). Highly efficient in practice.
- Use Cases: Very widely used due to its average-case efficiency. Often optimized with techniques like randomized pivot selection to mitigate worst-case scenarios.
6. Heapsort:
- Mechanism: Uses a heap data structure (a tree-based structure that satisfies the heap property: the value of each node is greater than or equal to the value of its children).
- Complexity: O(n log n) – guaranteed efficiency even in worst-case scenarios.
- Use Cases: Provides guaranteed O(n log n) performance, making it a good choice when predictable performance is crucial.
Applications of Sorting Technology:
Sorting algorithms are foundational to countless applications:
- Database Management Systems (DBMS): Indexing and querying data efficiently.
- Search Engines: Ranking search results.
- Data Visualization: Organizing data for charts and graphs.
- Operating Systems: Process scheduling, memory management.
- Machine Learning: Data preprocessing, feature engineering.
- Compiler Optimization: Optimizing code execution.
- Network Routing: Efficient packet routing.
Choosing the Right Algorithm:
Selecting the optimal sorting algorithm requires careful consideration of the specific context. Factors to consider include:
- Dataset size: For small datasets, simpler algorithms like insertion sort might suffice. For large datasets, merge sort or quicksort are typically preferred.
- Data characteristics: If the data is nearly sorted, insertion sort can be very efficient.
- Memory constraints: Some algorithms require significant extra memory (e.g., merge sort), while others operate in-place (e.g., quicksort).
- Stability: If the relative order of equal elements needs to be preserved, a stable sort (like merge sort) is necessary.
Conclusion:
Sorting technology is a cornerstone of computer science, underpinning many critical applications. Understanding the characteristics of different algorithms and their complexities is essential for developing efficient and scalable software systems. The choice of the best sorting algorithm is a crucial optimization step in numerous software development projects.
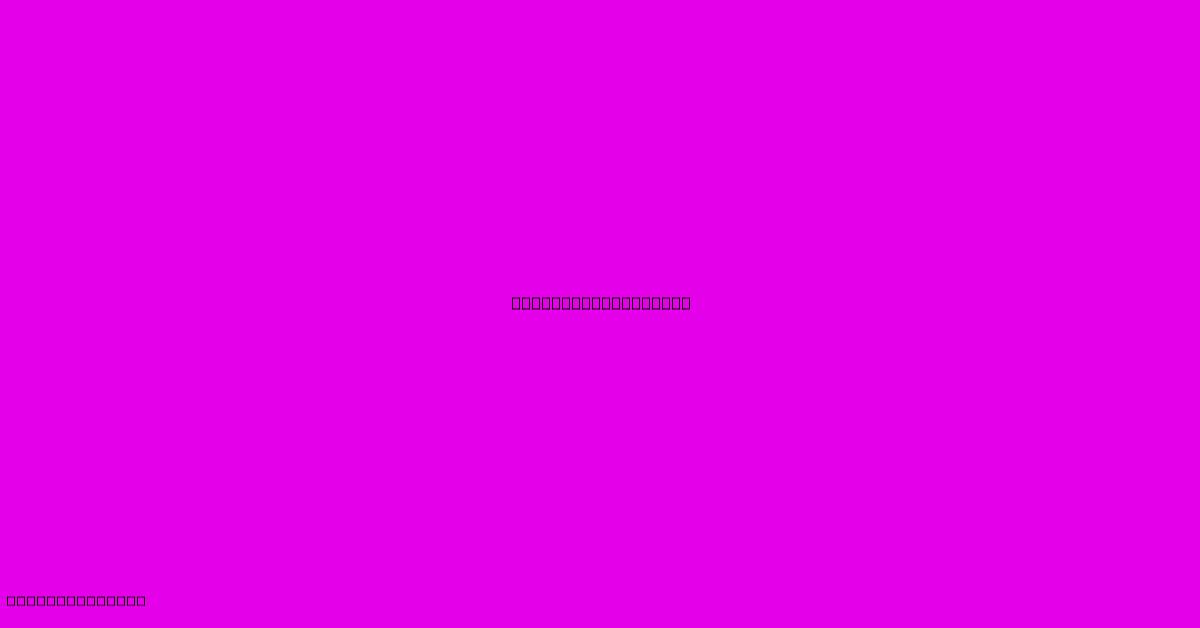
Thank you for visiting our website wich cover about Sorting Technology. We hope the information provided has been useful to you. Feel free to contact us if you have any questions or need further assistance. See you next time and dont miss to bookmark.
Featured Posts
-
Technology Designs
Jan 05, 2025
-
A Technological Advance Would Best Be Represented By
Jan 05, 2025
-
Technology Consulting Website Template
Jan 05, 2025
-
Idex Optical Technologies
Jan 05, 2025
-
Teijin Automotive Technologies Salisbury Nc
Jan 05, 2025