Jest Technologies
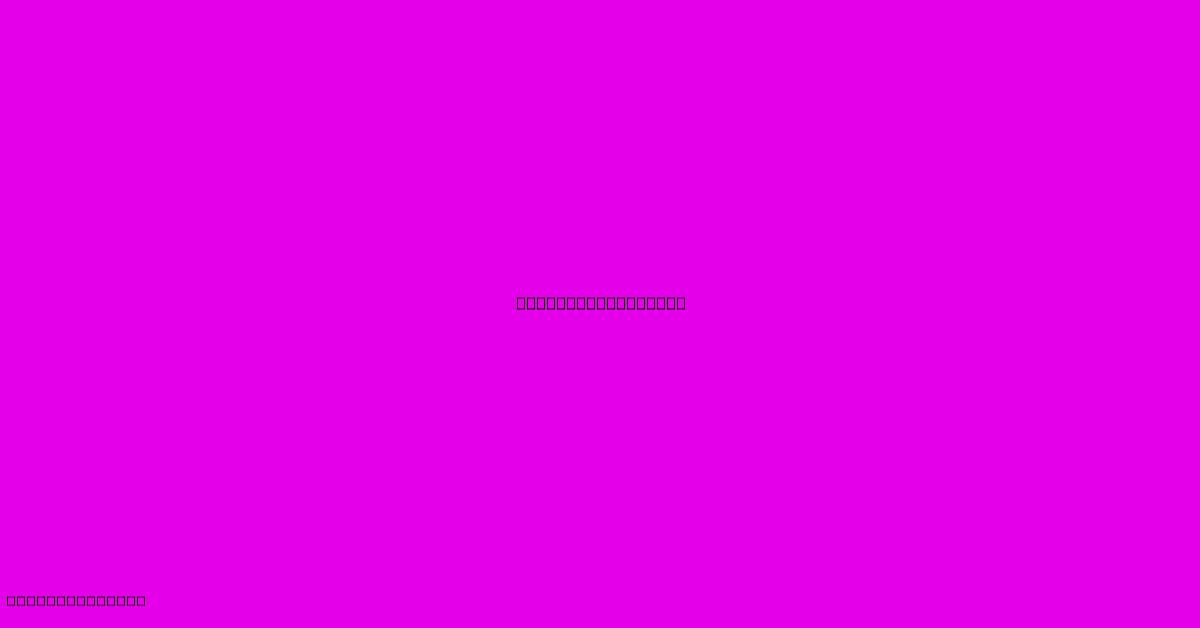
Discover more detailed and exciting information on our website. Click the link below to start your adventure: Visit Best Website mr.cleine.com. Don't miss out!
Table of Contents
Mastering Jest: Your Comprehensive Guide to JavaScript Testing
Jest, Facebook's powerful JavaScript testing framework, has become a staple in the developer's toolkit. Its ease of use, comprehensive features, and robust ecosystem make it an ideal choice for testing everything from small components to large-scale applications. This article dives deep into Jest, covering its core functionalities, advanced techniques, and best practices.
Why Choose Jest?
Jest's popularity stems from several key advantages:
-
Zero Configuration (Mostly): Getting started is a breeze. Jest requires minimal setup, often working out-of-the-box with simple projects. While configuration options exist for advanced scenarios, the initial learning curve is significantly lower than with many other testing frameworks.
-
Built-in Mocking: Jest provides excellent built-in mocking capabilities, simplifying the process of isolating units of code and simulating dependencies. This is crucial for writing reliable and maintainable tests.
-
Snapshot Testing: This unique feature allows you to capture the rendered output of components and compare it against previous snapshots. It's particularly useful for UI testing, ensuring consistency and catching unintended changes.
-
Watch Mode: Jest's watch mode automatically reruns tests whenever you make changes to your code, providing instant feedback and accelerating your development workflow.
-
Rich Ecosystem and Community: A vast community supports Jest, meaning plenty of resources, tutorials, and extensions are readily available.
Getting Started with Jest
The simplest way to start using Jest is by installing it via npm or yarn:
npm install --save-dev jest
or
yarn add --dev jest
After installation, you can typically run your tests with:
npm test
or
yarn test
(Assuming you've configured a test script in your package.json
file).
Writing Your First Jest Test
Let's create a simple function and test it:
sum.js
:
function sum(a, b) {
return a + b;
}
module.exports = sum;
sum.test.js
:
const sum = require('./sum');
test('adds 1 + 2 to equal 3', () => {
expect(sum(1, 2)).toBe(3);
});
This demonstrates a basic Jest test using the test
function and the expect
matcher. The toBe
matcher checks for strict equality. Jest offers a wide range of matchers for various comparison types.
Exploring Jest's Matchers
Jest provides a comprehensive set of matchers for various comparison needs:
toBe
: Strict equality (===)toEqual
: Deep equality (for objects and arrays)toBeTruthy
/toBeFalsy
: Checks for truthiness/falsinesstoContain
: Checks if an array contains a specific elementtoMatch
: Checks if a string matches a regular expressiontoThrow
: Checks if a function throws an error
Mocking with Jest
Mocking is crucial for isolating units of code and preventing dependencies from interfering with your tests. Jest's mocking capabilities make this process straightforward:
// mock.js
const fetchData = jest.fn(() => Promise.resolve({ data: 'mocked data' }));
module.exports = {fetchData};
// myComponent.test.js
const { fetchData } = require('./mock')
import myComponent from './myComponent';
test('component fetches data', async () => {
const result = await myComponent(fetchData);
expect(result).toEqual({data: 'mocked data'});
});
This example shows how to mock the fetchData
function, replacing its actual implementation with a mocked version that returns a promise resolving to specific data.
Advanced Jest Techniques
-
Setup and Teardown: Use
beforeEach
,afterEach
,beforeAll
, andafterAll
functions to perform setup and teardown actions before/after each test or all tests in a suite. -
Test Suites: Organize tests into suites using
describe
blocks to group related tests and improve readability. -
Asynchronous Testing: Use
async/await
or.then()
/.catch() to handle asynchronous operations in your tests. -
Custom Matchers: Create custom matchers to extend Jest's capabilities and handle specific testing scenarios.
Conclusion
Jest is a powerful and versatile testing framework that significantly enhances the development process. Its ease of use, comprehensive features, and strong community support make it a compelling choice for any JavaScript project. By mastering its core concepts and exploring its advanced techniques, you can build robust, reliable, and maintainable applications. Remember to leverage its features like mocking, snapshot testing, and watch mode to maximize efficiency and ensure the quality of your code.
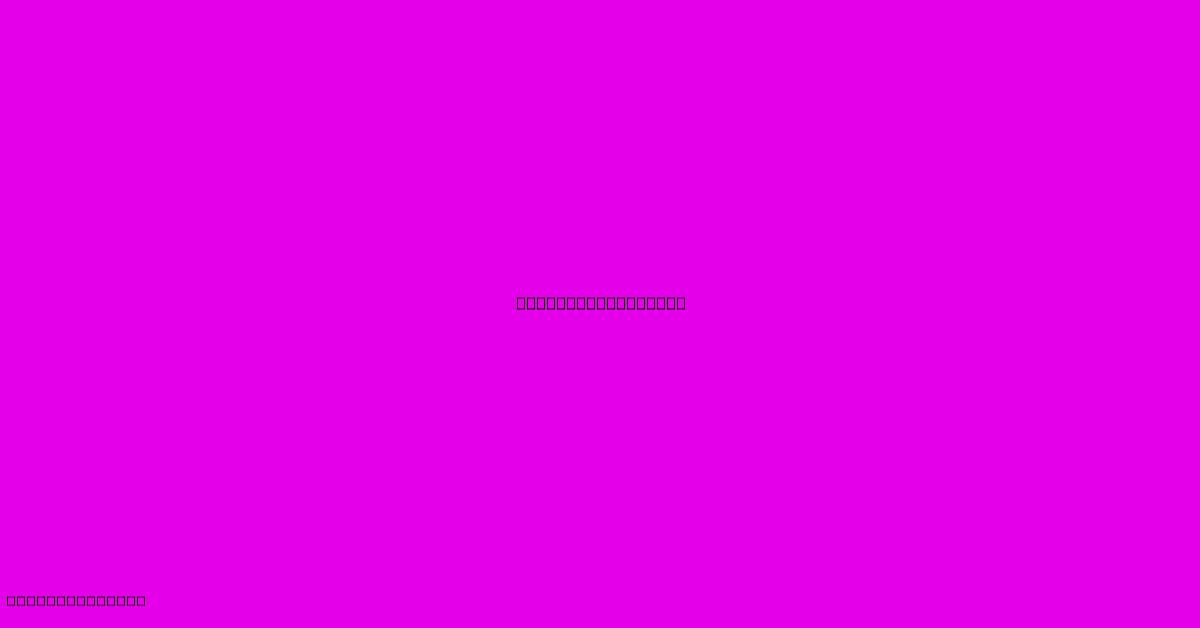
Thank you for visiting our website wich cover about Jest Technologies. We hope the information provided has been useful to you. Feel free to contact us if you have any questions or need further assistance. See you next time and dont miss to bookmark.
Featured Posts
-
Black Sage Technologies
Jan 04, 2025
-
Lattice Technology
Jan 04, 2025
-
Shippert Medical Technologies
Jan 04, 2025
-
United Technologies Corporation News
Jan 04, 2025
-
Define Geospatial Technology
Jan 04, 2025