Closure Technology
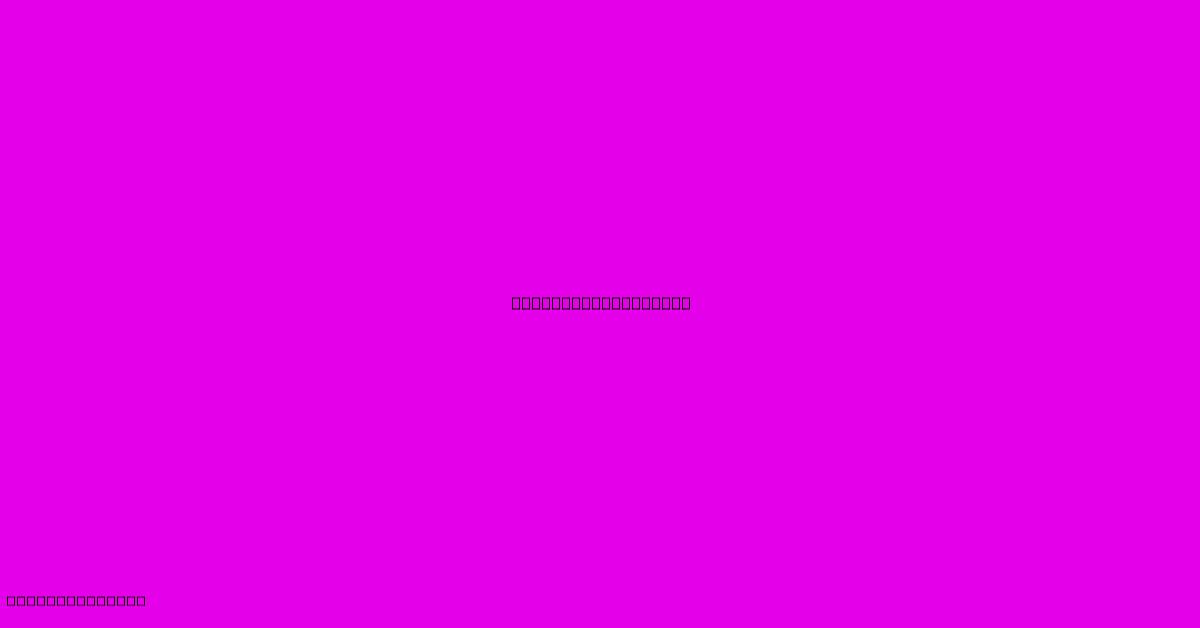
Discover more detailed and exciting information on our website. Click the link below to start your adventure: Visit Best Website mr.cleine.com. Don't miss out!
Table of Contents
Mastering Closure Technology: A Deep Dive into JavaScript's Powerful Feature
Closures are a fundamental concept in JavaScript that often mystify beginners but unlock powerful programming techniques for experienced developers. Understanding closures is crucial for writing efficient, maintainable, and elegant JavaScript code. This article provides a comprehensive overview of closure technology, demystifying its mechanics and showcasing its practical applications.
What is a Closure?
In essence, a closure is a function that has access to variables from its surrounding scope, even after that scope has finished executing. This "remembering" of its surrounding state is what makes closures so unique and powerful. Let's break this down:
-
Scope: Every function in JavaScript creates its own scope, a container for its variables. This is known as lexical scoping (or static scoping), meaning the scope is determined at the time the code is written, not at runtime.
-
Inner Function: A closure is formed when an inner function (a function defined inside another function) accesses variables from its outer function's scope. Even after the outer function has finished executing, the inner function retains access to these variables.
Example Illustrating a Closure:
function outerFunction() {
let outerVar = "Hello from outer!";
function innerFunction() {
console.log(outerVar);
}
return innerFunction;
}
let myClosure = outerFunction();
myClosure(); // Outputs: "Hello from outer!"
In this example, innerFunction
is a closure. Even though outerFunction
has already finished executing, innerFunction
still has access to outerVar
. This is because innerFunction
"closes over" the variables in its surrounding scope.
Key Benefits of Using Closures:
-
Data Encapsulation: Closures provide a way to create private variables. Variables declared within the outer function are inaccessible from outside, enhancing data protection and reducing the risk of accidental modification.
-
State Preservation: Closures allow functions to maintain state between invocations. This is invaluable for creating counters, timers, or any scenario where you need to track information across multiple function calls.
-
Currying and Partial Application: Closures are essential for implementing currying, a technique where a function is broken down into a series of functions, each taking a single argument. This improves code readability and reusability.
-
Enhancing Modular Design: Closures promote the creation of self-contained, modular code, leading to better organization and maintainability of larger JavaScript applications.
Common Use Cases:
-
Event Handling: Closures are extensively used in event handling. When an event listener is attached, it creates a closure that preserves the context of the event handler, even if the event triggers long after the listener is attached.
-
Asynchronous Programming: Closures are crucial in managing asynchronous operations (like AJAX calls) where the callback function needs to access variables from its surrounding scope.
-
Creating Private Members in Object-Oriented Programming: Closures facilitate the implementation of private members within objects, enforcing encapsulation and data hiding.
Potential Drawbacks:
While closures offer numerous advantages, it's important to be aware of potential downsides:
-
Memory Management: Since closures retain references to variables in their surrounding scope, they can potentially increase memory consumption if not managed carefully. Garbage collection will eventually reclaim this memory, but excessive closures could lead to performance issues.
-
Debugging Complexity: Debugging code that heavily relies on closures can be challenging, as the variable scope and lifetime become more intricate.
Conclusion:
Closures are a fundamental aspect of JavaScript programming, enabling powerful techniques for creating efficient and maintainable applications. While understanding their mechanics might require some initial effort, mastering closures is a significant step towards becoming a proficient JavaScript developer. By leveraging their capabilities, developers can build more robust, modular, and elegant JavaScript applications. Remember to carefully consider memory management to prevent potential performance issues.
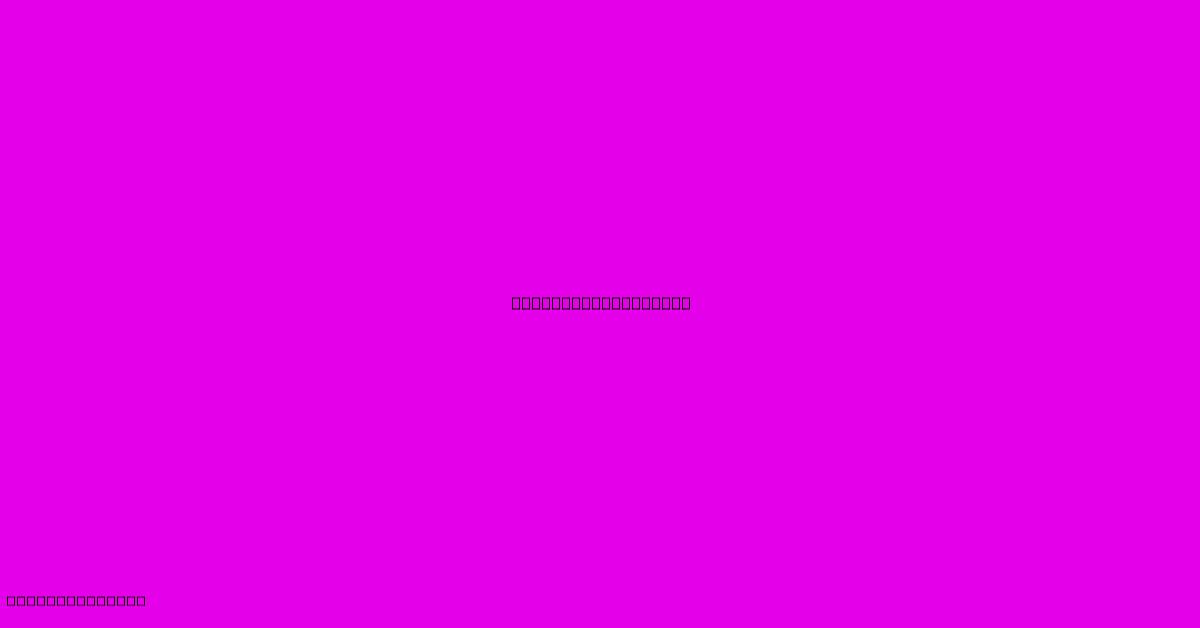
Thank you for visiting our website wich cover about Closure Technology. We hope the information provided has been useful to you. Feel free to contact us if you have any questions or need further assistance. See you next time and dont miss to bookmark.
Featured Posts
-
Trouville Stepson Triomphe De La
Dec 21, 2024
-
Atletico Vs Barcelona Perebutan Puncak Klasemen
Dec 21, 2024
-
Zobrazhennya Dodayte Relevantni Zobrazhennya Z Atributami Alt Scho Mistyat Klyuchovi Slova Tse Dopomozhe Pokraschiti Vizualne Spriynyattya Ta Seo
Dec 21, 2024
-
German Christmas Market Car Attack Casualties
Dec 21, 2024
-
Rey Misterio Sr Dead At 66
Dec 21, 2024